TCS CodeVita 2020 Interview Questions | Most Asked Questions of TCS CodeVita
TCS CodeVita 2020 Interview Questions
Q. 1 Consecutive Prime Sum
Some prime numbers can be expressed as a sum of other consecutive prime numbers. For example 5 = 2 + 3, 17 = 2 + 3 + 5 + 7, 41 = 2 + 3 + 5 + 7 + 11 + 13. Your task is to find out how many prime numbers which satisfy this property are present in the range 3 to N subject to a constraint that summation should always start with number 2.
Write code to find out the number of prime numbers that satisfy the above-mentioned property in a given range.
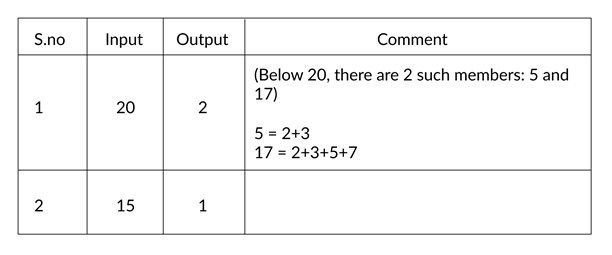
Input Format: The first line contains a number N
Output Format: Print the total number of all such prime numbers which are less than or equal to N.
Constraints: 2<N<=12,000,000,000
Sample Possible solution:
#include <stdio.h>
int prime(int b)
{
int j,cnt;
cnt=1;
for(j=2;j<=b/2;j++)
{
if(b%j==0)
cnt=0;
}
if(cnt==0)
return 1;
else
return 0;
}
int main() {
int i,j,n,cnt,a[25],c,sum=0,count=0,k=0;
scanf(“%d”,&n);
for(i=2;i<=n;i++)
{
cnt=1;
for(j=2;j<=n/2;j++)
{
if(i%j==0)
cnt=0;
}
if(cnt==1)
{
a[k]=i;
k++;
}
}
for(i=0;i<k;i++)
{
sum=sum+a[i];
c= prime(sum);
if(c==1)
count++;
}
printf(“%d”,count);
return 0;
}
Output:
20
2
Q 2. Counting Rock Samples
Juan Marquinho is a geologist and he needs to count rock samples in order to send it to a chemical laboratory. He has a problem: The laboratory only accepts rock samples by a range of its size in ppm (parts per million).
Juan Marquinho receives the rock samples one by one and he classifies the rock samples according to the range of the laboratory. This process is very hard because the number of rock samples may be in millions.
Juan Marquinho needs your help, your task is to develop a program to get the number of rocks in each of the ranges accepted by the laboratory.
This is an important question of TCS CodeVita 2020 Interview Questions
Input Format:
A positive integer S (the number of rock samples) separated by a blank space, and a positive integer R (the number of ranges of the laboratory); A list of the sizes of S samples (in ppm), as positive integers separated by space R lines where the ith line containing two positive integers, space-separated, indicating the minimum size and maximum size respectively of the ith range.
Output Format:
R lines where the ith line containing a single non-negative integer indicating the number of the samples which lie in the ith range.
Constraints:
10 ? S ? 10000 1 ? R ? 1000000 1?size of each sample (in ppm) ? 1000
Example 1
Input: 10 2
345 604 321 433 704 470 808 718 517 811
300 350
400 700
Output:
2 4
Explanation:
There are 20 samples and 3 ranges. The samples are 921, 107 195. The ranges are 1-100, 50-600, and 1-1000. Note that the ranges are overlapping. The number of samples in each of the three ranges is 1, 12, and 20 respectively. Hence the three lines of the output are 1, 12, and 20.
Possible Solution:
#include
int main() {
int a[1000],s,i,j,t,l1,l2,c=0;
scanf(“%d”,&s);
scanf(“%d”,&t);
for(i=0;i<s;i++)
scanf(“%d”,&a[i]);
for(i=0;i<t;i++)
{
scanf(“%d %d”,&l1,&l2);
for(j=0;j<s;j++)
{
if((a[j]>=l1)&&(a[j]<=l2))
c++;
}
printf(“%dn “,c);
c=0;
}
return 0;
}
Q 3. Bank Compare
There are two banks – Bank A and Bank B. Their interest rates vary. You have received offers from both banks in terms of the annual rate of interest, tenure, and variations of the rate of interest over the entire tenure. You have to choose the offer which costs you the least interest and rejects the other. Do the computation and make a wise choice.
The loan repayment happens at a monthly frequency and Equated Monthly Installment (EMI) is calculated using the formula given below :
EMI = loanAmount * monthlyInterestRate / ( 1 – 1 / (1 + monthlyInterestRate)^(numberOfYears * 12))
Constraints:
1 <= P <= 1000000
1 <=T <= 50
1<= N1 <= 30
1<= N2 <= 30
Input Format:
- First line: P principal (Loan Amount)
- Second line: T Total Tenure (in years).
- Third Line: N1 is the number of slabs of interest rates for a given period by Bank A. First slab starts from the first year and the second slab starts from the end of the first slab and so on.
- Next N1 line will contain the interest rate and their period.
- After N1 lines we will receive N2 viz. the number of slabs offered by the second bank.
- Next N2 lines are the number of slabs of interest rates for a given period by Bank B. The first slab starts from the first year and the second slab starts from the end of the first slab and so on.
- The period and rate will be delimited by a single white space.
Output Format: Your decision either Bank A or Bank B.
Explanation:
Example 1
Input
10000
20
3
5 9.5
10 9.6
5 8.5
3
10 6.9
5 8.5
5 7.9
Output:
Bank B
Example 2
Input
500000
26
3
13 9.5
3 6.9
10 5.6
3
14 8.5
6 7.4
6 9.6
Output: Bank A
Possible solution:
#include
#includeint main() {
double p,s,mi,sum,emi,bank[5],sq;
int y,n,k,i,yrs,l=0;
scanf(“%lf”,&p);
scanf(“%d”,&y);
for(k=0;k<2;k++)
{
scanf(“%d”,&n);
sum=0;
for(i=0;i<n;i++)
{
scanf(“%d”,&yrs);
scanf(“%lf”,&s);
mi=0;
sq=pow((1+s),yrs*12);
emi= (p*(s))/(1-1/sq);
sum= sum + emi;
}bank[l++]=sum;
}
if(bank[0]<bank[1])
printf(“Bank A”);
else
printf(“Bank B”);
return 0;
}
Q 4. The kth largest factor of N
A positive integer d is said to be a factor of another positive integer N if when N is divided by d, the remainder obtained is zero. For example, for number 12, there are 6 factors 1, 2, 3, 4, 6, 12. Every positive integer k has at least two factors, 1 and the number k itself. Given two positive integers N and k, write a program to print the kth largest factor of N.
Input Format: The input is a comma-separated list of positive integer pairs (N, k)
Output Format: The kth highest factor of N. If N does not have k factors, the output should be 1.
Constraints: 1<N<10000000000. 1<k<600. You can assume that N will have no prime factors which are larger than 13.
Example 1
- Input: 12,3
- Output: 4
Explanation: N is 12, k is 3. The factors of 12 are (1,2,3,4,6,12). The highest factor is 12 and the third-largest factor is 4. The output must be 4
Example 2
- Input: 30,9
- Output: 1
Explanation: N is 30, k is 9. The factors of 30 are (1,2,3,5,6,10,15,30). There are only 8 factors. As k is more than the number of factors, the output is 1.
Possible Solution:
#include <stdio.h>
int main() {
int n,k,i,c=0;
scanf(“%d”,&n);
scanf(“%d”,&k);
for(i=n;i>=1;i–)
{
if((n%i)==0)
c++;
if(c==k)
{
printf(“%d”,i);
break;
}
}
if(c!=k)
printf(“1”);
return 0;
}
Q 5. Football League
Football League Table Statement: All major football leagues have big-league tables. Whenever a new match is played, the league table is updated to show the current rankings (based on Scores, Goals For (GF), Goals Against (GA)). Given the results of a few matches among teams, write a program to print all the names of the teams in ascending order (Leader at the top and Laggard at the bottom) based on their rankings.
Rules: A win results in 2 points, a draw results in 1 point and a loss is worth 0 points. The team with the most goals in a match wins the match. Goal Difference (GD) is calculated as Goals For (GF) Goals Against (GA). Teams can play a maximum of two matches against each other Home and Away matches respectively.
The ranking is decided as follows: Team with maximum points is ranked 1 and minimum points are placed last Ties are broken as follows Teams with the same points are ranked according to Goal Difference(GD).
If Goal Difference(GD) is the same, the team with higher Goals For is ranked ahead
If GF is the same, the teams should be at the same rank but they should be printed in case-insensitive alphabetic according to the team names. More than 2 matches of the same teams, should be considered as Invalid Input.
A team can’t play matches against itself, hence if team names are same for a given match, it should be considered Invalid Input
This is an important question of TCS CodeVita 2020 Interview Questions
Input Format: The first line of input will contain a number of teams (N) the Second line contains names of the teams (Na) delimited by a whitespace character Third line contains a number of matches (M) for which results are available Next M lines contain a match information tuple {T1 T2 S1 S2}, where the tuple is comprised of the following information
- T1 Name of the first team
- T2 Name of the second team
- S1 Goals scored by the first team
- S2 Goals scored by the second team
Output Format: Team names in order of their rankings, one team per line OR Print “Invalid Input” where appropriate.
Constraints: 0< N <=10,000 0<=S1,S2
Example: Consider 5 teams Spain, England, France, Italy, and Germany with the following fixtures:
- Match 1: Spain vs. England (3-0) (Spain gets 2 points, England gets 0)
- Match 2: England vs. France (1-1) (England gets 1 point, France gets 1)
- Match 3: Spain vs. France (0-2) (Spain gets 0 points, France gets 2)
Table 1. Points Table after 3 matches
Since, Italy and Germany are tied for points, goals difference is checked. Both have the same, so, Goals For is checked. Since both are the same. Germany and Italy share the 4th rank. Since Germany appears alphabetically before Italy, Germany should be printed before Italy. Then the final result is: France Spain England Germany Italy
Sample:
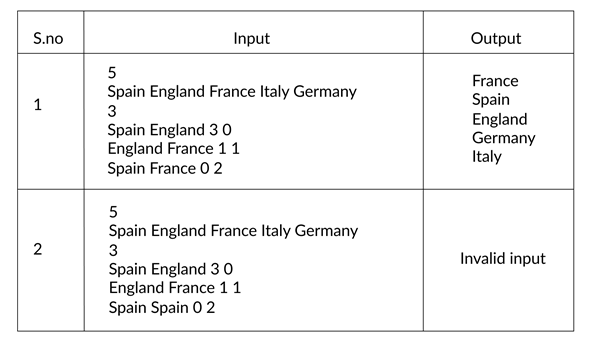
Registration Link for TCS Codevita 2020: Click here
If You Want To Get More Daily Such Jobs Updates, Career Advice Then Join the Telegram Group From Given Link And Never Miss Update.
Join Telegram Group of Daily Jobs Updates for 2010-2021 Batch: Click Here
Why You’re Not Getting Response From Recruiter?: Click here
How To Get a Job Easily: Professional Advice For Job Seekers: Click here
Cognizant Latest News: Up To 20K+ Employees Will Be Hired: Click here
COVID-19 Live Tracker India & Coronavirus Live Update: Click here
Why Remove China Apps took down from Play store?: Click here
Feel Like Demotivated? Check Out our Motivation For You: Click here
List of Best Sites To Watch Free Movies Online in 2020: Click here
5 Proven Tips For How To Look Beautiful and Attractive: Click here